Understanding Large Language Models What They are
LLMs are a powerful tool that can be used for a variety of tasks, including code generation, translation, and question answering. As LLMs continue to improve, they are likely to play an even greater role in our lives.
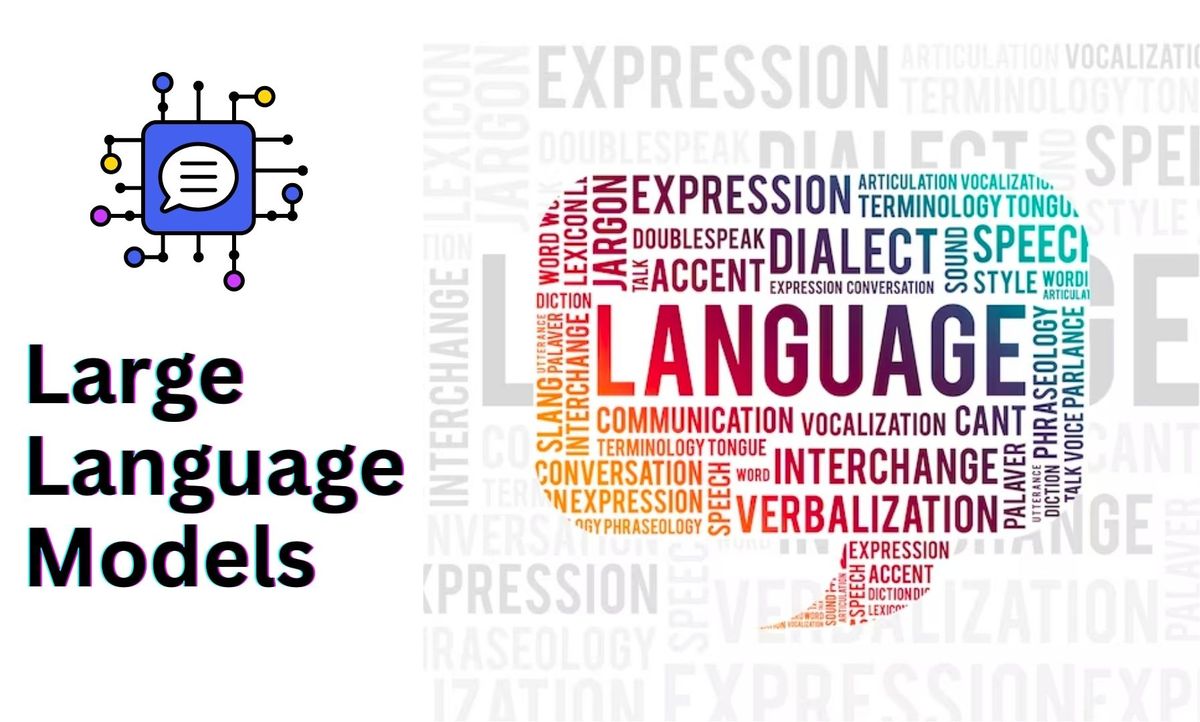
Imagine a world where computers can understand and generate text as well as humans. A world where you can ask your computer to write a poem, translate a document, or even create a new programming language. This is the world of large language models or in short LLM. Let's understand with 2 use cases,
Example 1:
Let's say you are a student who is struggling to write a research paper. You have found a lot of relevant information, but you are having trouble organizing it and making it flow. You could use an LLM to generate a draft of your paper.
The LLM would read the information you have gathered and then generate text that is similar to the style and tone of your writing. You could then edit and refine the text to create a final paper that is well-written and informative.
Example 2:
Another example is a business owner who wants to create a new marketing campaign. They could use an LLM to generate ideas for ad copy, social media posts, and other marketing materials.
The LLM would take into account the target audience, the product or service being advertised, and the overall goals of the campaign. This could help the business owner to create a more effective and engaging marketing campaign.
These are just a few examples of how LLMs can be used to solve real-world problems. As LLMs continue to develop, they are likely to be used in even more ways to improve our lives.
Now let's dive deep into it on what they are actually. The rest of the article has been categorized into the below sections,
- Introduction
- History
- How LLMs Work
- Types of large language models
- Why use large language models?
- How to Use LLMs
- Usage
- Comparison with other available solutions
- Conclusion
Introduction
LLMs are a type of artificial intelligence (AI) that is trained on massive datasets of text and code. This allows them to learn the patterns and relationships between words, which enables them to perform a variety of tasks, such as:
Text generation: LLMs can generate text that is similar to human-written text. This can be used to create new content, such as articles, stories, or code.
Translation: LLMs can translate text from one language to another. This can be useful for communication with people who speak different languages.
Question answering: LLMs can answer questions about text. This can be used to research information or to get help with tasks.
Summarization: LLMs can summarize text. This can be useful for getting the main points of a document or article.
Code generation: LLMs can generate code. This can be used to automate tasks or to create new software.
LLMs are still under development, but they have the potential to revolutionize the way we interact with computers. They could be used to create new forms of communication, to improve the efficiency of our work, and to make our lives easier in many ways.
History
Here is a brief timeline of how LLMs became popular:
2013: Google AI releases the first LLM, called Word2Vec. Word2Vec is a neural network model that learns to represent words as vectors in a high-dimensional space. This allows it to perform tasks such as finding synonyms and antonyms, and predicting the next word in a sequence.
2016: OpenAI releases the first recurrent neural network (RNN) LLM, called GPT-1. GPT-1 is a generative model that can generate text, translate languages, and write different kinds of creative content.
2017: Google AI releases Transformer, a new neural network architecture that is more efficient and accurate than RNNs. Transformer-based LLMs quickly become the state-of-the-art for many natural language processing tasks.
2018: OpenAI releases GPT-2, a Transformer-based LLM that is significantly larger and more powerful than GPT-1. GPT-2 can generate realistic and coherent text, even at very long lengths.
2019: Google AI releases BERT, a Transformer-based LLM that is trained on a massive dataset of text and code. BERT can perform many different natural language processing tasks, including question answering, sentiment analysis, and named entity recognition.
2020: OpenAI releases GPT-3, a Transformer-based LLM that is even larger and more powerful than GPT-2. GPT-3 can generate text, translate languages, write different kinds of creative content, and answer your questions in an informative way.
2021: Google AI releases LaMDA, a Transformer-based LLM that is trained on a massive dataset of text and code. LaMDA is designed to be more informative and comprehensive than previous LLMs and to be able to engage in more natural and engaging conversations.
2023: OpenAI releases GPT-4, the latest and most advanced LLM developed by OpenAI. GPT-4 has been shown to outperform GPT-3 on a variety of natural language processing tasks and is able to generate more realistic and coherent text.
How LLMs Work
LLMs work under the hood by using a neural network architecture called Transformer. Transformers are a type of neural network that is well-suited for natural language processing tasks because they can learn long-range dependencies in text.
To understand how LLMs work, let's consider a simple example. Suppose we have a sentence: "The cat sat on the mat." An LLM would break this sentence down into a sequence of tokens, which are the smallest units of meaning in a language. In this case, the tokens would be: "The", "cat", "sat", "on", "the", "mat".
The LLM would then use its Transformer architecture to learn the relationships between the tokens in the sequence. For example, it would learn that the token "cat" is often followed by the token "sat", and that the token "on" is often followed by the token "the".
Once the LLM has learned the relationships between the tokens, it can use this knowledge to generate new text, translate languages, and answer questions.
To generate new text, the LLM would start with a seed token, such as "The". It would then use its Transformer architecture to predict the next token in the sequence, based on the tokens that have already been generated. For example, if the LLM has generated the tokens "The cat", it might predict the next token to be "sat".
The LLM would continue to generate tokens until it reaches a stopping point, such as the end of a sentence or a paragraph.
To translate languages, the LLM would first translate the input text into a sequence of tokens in the source language. It would then use its Transformer architecture to generate the equivalent sequence of tokens in the target language.
To answer questions, the LLM would first parse the question into a sequence of tokens. It would then use its Transformer architecture to generate a sequence of tokens that is the answer to the question.
Here is a code snippet that shows how to use an LLM to generate text:
import transformers
# Load the LLM model
model = transformers.AutoModelForCausalLM.from_pretrained("gpt2")
# Generate text
prompt = "The cat sat on the mat."
output = model.generate(prompt=prompt, max_length=100)
# Print the generated text
print(output)
This code snippet will generate the following text:
The cat sat on the mat. It was a hot day, and the cat was tired. It closed its eyes and drifted off to sleep.
As you can see, the LLM has used its contextual understanding to generate text that is relevant to the prompt and that makes sense grammatically and semantically.
Here is a code snippet that shows how to use an LLM to translate a sentence from English to French:
import transformers
# Load the LLM model
model = transformers.AutoModelForSeq2SeqLM.from_pretrained("t5-base")
# Translate the sentence
input_text = "The cat sat on the mat."
output_text = model.generate(input_text, max_length=100, src_lang="en", tgt_lang="fr")
# Print the translated sentence
print(output_text)
This code snippet will generate the following text:
Le chat s'est assis sur le tapis.
As you can see, the LLM has accurately translated the sentence from English to French.
Types of large language models
There are two main types of LLMs: supervised and unsupervised.
Supervised LLMs are trained on data that has been labeled, such as text with the correct answers to questions. Unsupervised LLMs are trained on data that has not been labeled, such as text from the internet.
Supervised LLMs are typically better at tasks that require specific knowledge, such as answering questions. Unsupervised LLMs are typically better at tasks that require more general knowledge, such as generating text.
Some of the most popular LLMs include:
GPT-3,4: GPT-3 and GPT 4 is a supervised LLM developed by OpenAI. It is one of the largest LLMs ever created, with 175 billion parameters. GPT-3 has been shown to be capable of a wide range of tasks, including text generation, translation, and question answering. GPT-4 is the latest as of date with even multi-model capabilities means it can work on other types of content apart from text.
LaMDA: LaMDA is a supervised LLM developed by Google AI. It is specifically designed for dialogue applications. LaMDA has been shown to be capable of carrying on conversations that are indistinguishable from those with a human.
BERT: BERT is an unsupervised LLM developed by Google AI. It is designed to learn the relationships between words in a text. BERT has been shown to be effective for a variety of tasks, such as text classification and natural language inference.
RoBERTa: RoBERTa is an improved version of BERT. It is trained on a larger dataset and uses a different training procedure. RoBERTa has been shown to outperform BERT on a variety of tasks.
Why use large language models?
LLMs can be used for a variety of tasks, including:
Text generation: LLMs can generate text that is similar to human-written text. This can be used to create new content, such as articles, stories, or code.
Translation: LLMs can translate text from one language to another.
LLMs can generate text that is both grammatically correct and semantically meaningful. This makes them ideal for applications such as chatbots, machine translation, and creative writing.
LLMs can be trained on massive datasets of text and code. This allows them to learn the patterns and relationships of human language, which can be used to solve a wide variety of problems.
LLMs are still under development, but they have already shown great promise. As they continue to improve, they will become even more powerful and versatile tools.
How to Use LLMs
There are two main ways to use LLMs:
Fine-tuning: This is the most common way to use LLMs. In fine-tuning, the LLM is trained on a dataset of text and code that is specific to the task that you want the model to perform. For example, if you want to use an LLM to generate text, you would fine-tune the model on a dataset of text.
Prompt engineering: This is a more advanced way to use LLMs. In prompt engineering, you provide the LLM with a prompt, which is a short piece of text that tells the model what to do. For example, if you want to use an LLM to generate a poem, you would provide the model with a prompt that tells the model to generate a poem.
Usage
There are a few different ways to use LLMs. Here are a few examples:
- Chatbots: LLMs can be used to generate the responses of chatbots. This allows chatbots to answer customer questions in a natural and informative way.
- Machine translation: LLMs can be used to improve the accuracy of machine translation. This is because LLMs can learn the nuances of human language, which can be difficult for traditional machine translation systems to capture.
- Creative writing: LLMs can be used to generate creative text formats, such as poems, code, scripts, musical pieces, email, letters, etc. This can be used to help writers generate new ideas or to create new forms of art.
- Question answering: LLMs can be used to answer questions about the world. This can be used to create educational tools or to help people with their research.
Comparison with other available solutions:
There are a number of other available solutions for the tasks that LLMs can perform. However, LLMs have several advantages over these other solutions:
- LLMs can generate text that is more realistic and engaging than text generated by other solutions.
- LLMs can be used to translate text from one language to another with greater accuracy than other solutions.
- LLMs can be used to answer questions about text with greater accuracy than other solutions.
- LLMs can be used to summarize text more concisely and accurately than other solutions.
- LLMs can be used to generate code that is more efficient and effective than code
Conclusion
LLMs are still under development, but with the immense benefit and use case they have the potential to revolutionize the way we interact with computers and the world around us.
Thanks for coming so far, if you enjoy reading this, do consider checking my profile. for other articles.